Code
import plotnine
print(plotnine.__version__)
plotnine is an implementation of a grammar of graphics in Python based on ggplot2.
make 3 plot per row
find all build in themes in https://github.com/has2k1/plotnine/tree/main/plotnine/themes
new_data=dowjones4.sample(50, random_state=42)
new_data=new_data.sort_values(by=['Date'], ascending=True)
#write a function that creates all the plots
def plot(x):
df2 = dowjones4.query('Date <= @x')
p = (ggplot(df2,
aes(x = 'Date', y = 'Price'))
+ geom_line(aes(color="type"))
# Specify the limits for the x and y aesthetics
#+ scale_x_continuous(limits=(dowjones4.Date.min(), dowjones4.Date.max()))
#+ scale_y_continuous(limits=(dowjones4.Price.min(), dowjones4.Price.max()))
+ theme(subplots_adjust={'right': 0.85}) # Make space for the legend
)
return(p)
plots = (plot(i) for i in (new_data["Date"]))
https://plotnine.org/
---
title: "plotnine chart"
execute:
warning: false
error: false
eval: false
format:
html:
toc: true
toc-location: right
code-fold: show
code-tools: true
number-sections: true
code-block-bg: true
code-block-border-left: "#31BAE9"
---
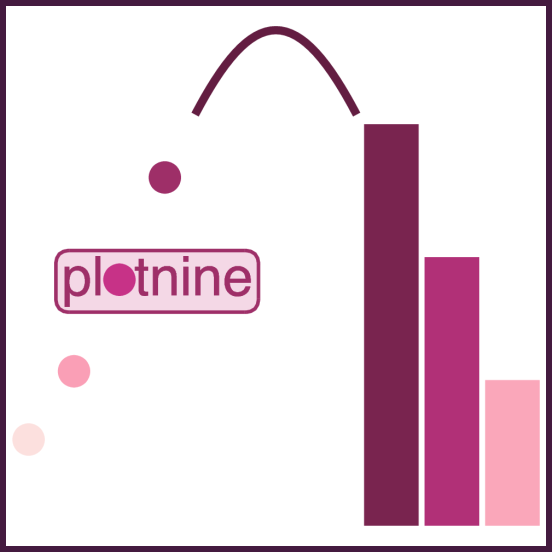{width="300"}
plotnine is an implementation of a grammar of graphics in Python based on ggplot2.
```{python}
import plotnine
print(plotnine.__version__)
```
```{python}
from plotnine import *
print(plotnine.__version__)
```
```{python}
from plotnine import *
import seaborn as sns
import pandas as pd
# Apply the default theme
# Load an example dataset
tips = sns.load_dataset("tips")
tips.head()
```
# Scatter Plot
```{python}
p=(
ggplot(data=tips)+aes(x="tip",y="total_bill")+ geom_point()
)
p
```
## color by group
```{python}
p=(
ggplot(data=tips)+aes(x="tip",y="total_bill")+ geom_point(aes(color="sex"))
)
p
```
## size by group
```{python}
p=(
ggplot(data=tips)+aes(x="tip",y="total_bill",size="size")+ geom_point()
)
p
```
# line Plot
```{python}
dowjones= sns.load_dataset("dowjones")
dowjones.head()
```
```{python}
p=(
ggplot(data=dowjones)+aes(x="Date",y="Price")+ geom_line()
)
p
```
```{python}
#| code-fold: true
import random
from siuba import _, mutate, filter, group_by, summarize,show_query
from siuba import *
dowjones2=dowjones>>mutate(type='old')
dowjones3=dowjones>>mutate(Price=_.Price+random.random()*200,type='new')
dowjones4=pd.concat([dowjones2, dowjones3], ignore_index = True)>> arrange(_.Date)
```
```{python}
dowjones4.head()
```
## color by group
```{python}
p=(
ggplot(data=dowjones4)+aes(x="Date",y="Price")+ geom_line(aes(color="type"))
)
p
```
# histogram
```{python}
p=(
ggplot(data=tips)+aes(x="tip")+ geom_histogram()
)
p
```
## color by group
```{python}
p=(
ggplot(data=tips)+aes(x="tip",fill = 'sex')+ geom_histogram(position = 'dodge')
)
p
```
# bar chart
```{python}
p=(
ggplot(data=tips)+aes(x='sex',y='tip',fill="sex")+geom_col()
)
p
```
# box plot
```{python}
p=(
ggplot(data=tips)+aes(x='day',y='tip',fill="day")+geom_boxplot()
)
p
```
## color by group
```{python}
p=(
ggplot(data=tips)+aes(x='day',y='tip',fill="sex")+geom_boxplot()
)
p
```
# strip plot
```{python}
p=(
ggplot(data=tips)+aes(x='day',y='tip')+geom_jitter(width=0.1)
)
p
```
## color by group
```{python}
p=(
ggplot(data=tips)+aes(x='day',y='tip',fill="sex")+geom_jitter(position=position_jitterdodge())
)
p
```
# Facet plot
```{python}
p=(
ggplot(data=tips)+aes(x="tip",y="total_bill")+ geom_point(aes(color="sex"))
+ facet_wrap("day")
)
p
```
make 3 plot per row
```{python}
p=(
ggplot(data=tips)+aes(x="tip",y="total_bill")+ geom_point(aes(color="sex"))
+ facet_wrap("day",ncol = 3)
)
p
```
# title,size, x y names
## add title
```{python}
p=(
ggplot(data=tips)+aes(x="tip",fill = 'sex')+ geom_histogram(position = 'dodge')+ ggtitle("tip by sex")
)
p
```
## adjust size
```{python}
p=(
ggplot(data=tips)+aes(x="tip",fill = 'sex')+ geom_histogram(position = 'dodge')+ ggtitle("tip by sex")+ theme(figure_size=(4, 3))
)
p
```
## change x y name
```{python}
p=(
ggplot(data=tips)+aes(x="tip",y="total_bill")+ geom_point()+ scale_x_continuous(name="new x name")+ scale_y_continuous(name="new y name")
)
p
```
# applying themes
find all build in themes in https://github.com/has2k1/plotnine/tree/main/plotnine/themes
::: {.panel-tabset .nav-pills}
## xkcd
```{python}
p=(
ggplot(data=tips)+aes(x="tip",fill = 'sex')+ geom_histogram(position = 'dodge')+ theme_xkcd()
)
p
```
## theme_538
```{python}
p=(
ggplot(data=tips)+aes(x="tip",fill = 'sex')+ geom_histogram(position = 'dodge')+ theme_538()
)
p
```
## theme_dark
```{python}
p=(
ggplot(data=tips)+aes(x="tip",fill = 'sex')+ geom_histogram(position = 'dodge')+ theme_dark()
)
p
```
:::
# Save plot
```{python}
p=(
ggplot(data=tips)+aes(x="tip",fill = 'sex')+ geom_histogram(position = 'dodge')+ theme_dark()
)
p.save(filename = 'test3.png')
```
# Animation plot
```{python}
from plotnine.animation import PlotnineAnimation
```
```{python}
#| output: false
new_data=dowjones4.sample(50, random_state=42)
new_data=new_data.sort_values(by=['Date'], ascending=True)
#write a function that creates all the plots
def plot(x):
df2 = dowjones4.query('Date <= @x')
p = (ggplot(df2,
aes(x = 'Date', y = 'Price'))
+ geom_line(aes(color="type"))
# Specify the limits for the x and y aesthetics
#+ scale_x_continuous(limits=(dowjones4.Date.min(), dowjones4.Date.max()))
#+ scale_y_continuous(limits=(dowjones4.Price.min(), dowjones4.Price.max()))
+ theme(subplots_adjust={'right': 0.85}) # Make space for the legend
)
return(p)
plots = (plot(i) for i in (new_data["Date"]))
```
```{python}
from matplotlib import rc
rc("animation", html="html5")
#create the animation
animation = PlotnineAnimation(plots, interval=300, repeat_delay=500)
animation
```
# reference:
https://plotnine.org/