Code
=1
atype(a)
int
len()
count()
index()
Tony Duan
len()
count()
index()
show the first ‘apple’ index. python list start at 0
all ‘apple’ in the list
using loop:
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
using List Comprehensions
('orange', 'apple', 'pear', 'banana', 'kiwi', 'apple', 'banana', 'apple')
tuple can not be modified.
A set is an unordered collection with no duplicate elements.
{'apple', 'banana', 'orange', 'pear'}
NumPy is the fundamental package for scientific computing in Python. It is a Python library that provides a multidimensional array object
Python doesn’t have a built-in type for matrices. However, we can treat a list of a list as a matrix
[[1, 4, 5, 12], [-5, 8, 9, 0], [-6, 7, 11, 19]]
Counter({np.int64(0): 2, np.int64(3): 1, np.int64(4): 1})
[[ 1 4 5 12]
[-5 8 9 0]
[-6 7 11 19]
[ 1 4 5 12]
[-5 8 9 0]
[-6 7 11 19]
[ 1 4 5 12]
[-5 8 9 0]
[-6 7 11 19]]
array([[False, False, True, True],
[False, True, True, False],
[False, True, True, True],
[False, False, True, True],
[False, True, True, False],
[False, True, True, True],
[False, False, True, True],
[False, True, True, False],
[False, True, True, True]])
array([[ 1, 4, 5, 12],
[-5, 8, 9, 0],
[-6, 7, 11, 19],
[ 1, 4, 5, 12],
[-5, 8, 9, 0],
[-6, 7, 11, 19],
[ 1, 4, 5, 12],
[-5, 8, 9, 0],
[-6, 7, 11, 19]])
filter secound column>5
which only keep 2,3 row.
https://docs.python.org/3/tutorial/datastructures.html#
https://numpy.org/doc/stable/user/basics.rec.html
---
title: "Data structure in Python"
author: "Tony Duan"
execute:
warning: false
error: false
format:
html:
toc: true
toc-location: right
code-fold: show
code-tools: true
number-sections: true
code-block-bg: true
code-block-border-left: "#31BAE9"
---
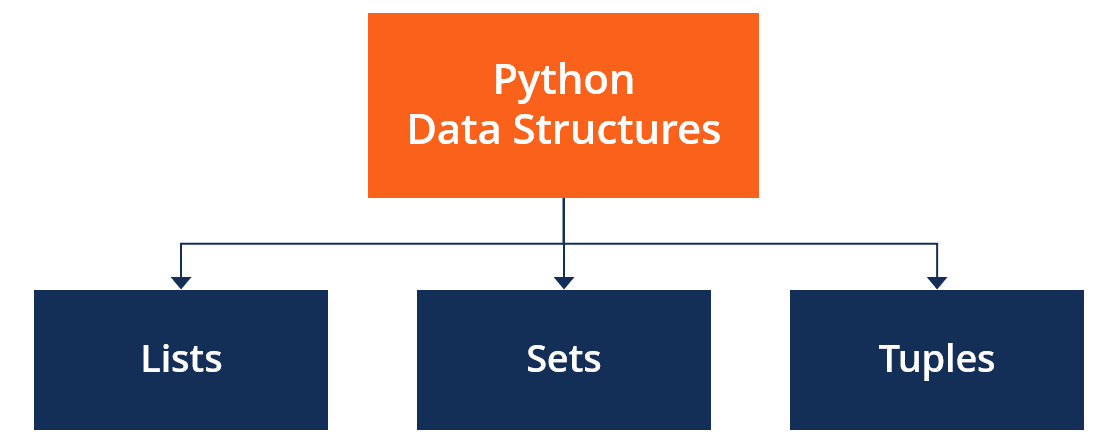{width="500"}
# bulid-in data Structures
## singular
```{python}
a=1
type(a)
```
```{python}
a=1.3
type(a)
```
```{python}
a='hell'
type(a)
```
```{python}
a= True
type(a)
```
## list
```{python}
a=[1,2,3]
a
```
```{python}
type(a)
```
```{python}
fruits = ['orange', 'apple', 'pear', 'banana', 'kiwi', 'apple', 'banana','apple']
```
### find length of the list with `len()`
```{python}
len(fruits)
```
### first 2 on the list
```{python}
fruits[:2]
```
### last 2 on the list
```{python}
fruits[-2:]
```
### find how many time in the list with `count()`
```{python}
fruits.count('apple')
```
### find locaiton of on the list with `index()`
show the first 'apple' index. python list start at 0
```{python}
fruits.index('apple')
```
all 'apple' in the list
```{python}
[index for index, value in enumerate(fruits) if value == 'apple']
```
### reverse the list
```{python}
fruits.reverse()
fruits
```
### sort the list
```{python}
fruits.sort()
fruits
```
### add element on the list
```{python}
fruits.append('grape')
fruits
```
### drop last element
```{python}
fruits.pop()
fruits
```
### List Comprehensions
using loop:
```{python}
squares = []
for x in range(10):
squares.append(x**2)
squares
```
using List Comprehensions
```{python}
squares = [x**2 for x in range(10)]
squares
```
### list to Tuples
```{python}
tuple(squares)
```
### list to set
```{python}
set(squares)
```
### list to dictionary
#### one list to dictionary
```{python}
list=['a', 1, 'b', 2, 'c', 3]
def convert(lst):
res_dict = {}
for i in range(0, len(lst), 2):
res_dict[lst[i]] = lst[i + 1]
return res_dict
convert(list)
```
#### two list to dictionary
```{python}
import itertools
keys = ('name', 'age', 'food')
values = ('Monty', 42, 'spam')
dict(zip(keys, values))
```
## Tuples
```{python}
fruits = ('orange', 'apple', 'pear', 'banana', 'kiwi', 'apple', 'banana','apple')
fruits
```
```{python}
type(fruits)
```
tuple can not be modified.
## Sets
A set is an unordered collection with no duplicate elements.
```{python}
basket = {'apple', 'orange', 'apple', 'pear', 'orange', 'banana'}
basket
```
```{python}
type(basket)
```
## Dictionaries
```{python}
tel = {'jack': 4098, 'sape': 4139}
tel
```
```{python}
type(tel)
```
```{python}
tel['jack']
```
# numpy data Structures(matrix in python)
NumPy is the fundamental package for scientific computing in Python. It is a Python library that provides a multidimensional array object
Python doesn't have a built-in type for matrices. However, we can treat a list of a list as a matrix
```{python}
A = [[1, 4, 5, 12],
[-5, 8, 9, 0],
[-6, 7, 11, 19]]
A
```
## numpy Array
```{python}
import numpy as np
A2 = np.array([[1, 2, 3], [3, 4, 5]])
print(A2)
```
```{python}
type(A2)
```
## shape
```{python}
A2.shape
```
## row number
```{python}
len(A2)
```
## total elements
```{python}
A2.size
```
## dimension
```{python}
A2.ndim
```
## count numpy.ndarray
```{python}
import collections, numpy
a = numpy.array([0, 3, 0, 4])
collections.Counter(a)
```
### convert list into numpy array
```{python}
A = [
[1, 4, 5, 12],
[-5, 8, 9, 0],
[-6, 7, 11, 19],
[1, 4, 5, 12],
[-5, 8, 9, 0],
[-6, 7, 11, 19],
[1, 4, 5, 12],
[-5, 8, 9, 0],
[-6, 7, 11, 19]
]
A3 = np.array(A)
print(A3)
```
### selection
#### first 5 row
```{python}
A[:5]
```
#### lst 5 row
```{python}
A[:-5]
```
#### first row
```{python}
A[0]
```
#### first column
```{python}
A2[:,0]
```
#### first row and first column element
```{python}
A2[0,0]
```
```{python}
A2.dtype
```
#### 2 row and 3 column
```{python}
A2[1,2]
```
#### filter
##### filter all
```{python}
print(A3)
```
```{python}
A3>4
```
```{python}
A3[A3>4]
```
##### filter row
```{python}
A3
```
filter secound column>5
```{python}
filter_val=(A3>5)[:,2]
```
which only keep 2,3 row.
```{python}
A3[filter_val,0:]
```
### create numpy array
#### create eye
```{python}
np.eye(3)
```
#### create zero
```{python}
np.zeros((2,3))
```
#### create ones
```{python}
np.ones((2,3))
```
### compare
```{python}
# Creating Array
a = np.array([1,2,3,4])
b = np.array([3,2,5,6])
```
```{python}
# Comparing two arrays
np.greater(a, b)
```
```{python}
a >= b
```
```{python}
# Comparing two arrays
np.less(a, b)
```
```{python}
# Comparing two arrays
np.equal(a, b)
```
### reshape
```{python}
a=np.arange(9).reshape(3, 3)
a
```
### calculation
```{python}
b=a*a
b
```
```{python}
b=a+a
b
```
### numpy array to dataframe
```{python}
import pandas as pd
df = pd.DataFrame(b, columns=['Column_A', 'Column_B', 'Column_C'])
df
```
# pandas series
```{python}
```
# Reference
https://docs.python.org/3/tutorial/datastructures.html#
https://numpy.org/doc/stable/user/basics.rec.html